milestone:
final submission:
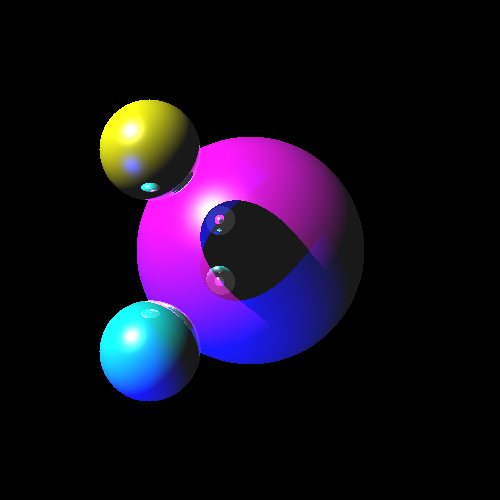
We've implemented all of everything that was asked of the assignment. Our solution varies on the edges from the feedback server and minor shading details from round off error.
Some important things to note is that for our output function, you must specify a .ppm file, for example, "output coolscene.ppm" because our raytracer writes out in .ppm format.
We've included the use of all geometries, including the trinormals with interpolated normals for shading and reflection.
Our transformations uses the stack matrix we developed back from assignment 2 -- which translates, rotates, scales, pushes, and pops.
Our lighting includes direcitonal, points, attentuation, shadowing. As well as reflection weighted from the specular.
We also implemented the Blinn-Phong shading model which incorporated the shadow detection and the reflections.
We built our own acceleration data structure that implemented Axis-Aligned Bounding Boxes. Our method was to create all the leaf nodes of primitives after parsing the file. Then we recursively build the tree from the root by passing in a vector of leaf nodes. The root splits the nodes by getting an average of either x, y, or z points from the center of each bounding box (x,y,z alternate every depth). Those that are less than the average, we recursively put into the left child and those that are more go into the right child. It does a rough partitioning based on the center component, so we can achieve smaller overall bounding boxes as the tree recurses down. We keep the tree balanced by ensuring that half of the nodes go into the left and the other half into the right. After building the tree, we can intersect each root with a ray. If the bounding box is hit, we recursively intersect the left and right child boxes, otherwise it will return a "Not Hit" message. We extract the min t by doing a simple comparson from what the left and right child return. The tree has log(base2)n depth, where n = number of primitives.
We also used OpenMP to allow two threads to run in parallel.
Some interesting runtimes with OpenMP, naively intersecting all objects, and AABB:
|
AABB w/ OpenMP |
AABB w/o OpenMP |
Naive w/o OpenMP |
scene4 |
7.35s |
12.21s |
10.03s |
scene5 |
12.12s |
14.06s |
54.07s |
scene6 |
34.41s |
67.91s |
38.38s |
scene7 |
107.35s |
194.29s |
>30min |
Extra Credit:
For extra credit, we implemented soft shadows using random sampling similar to that of a Monte Carlo method. We shoot many rays into a sphere around a point light. Then we weight the shadows by the number of rays that hit an object over all the total rays shot. Here is a progression of increasing the sampling rate on a scene:
10 rays
20 rays
30 rays
40 rays
50 rays
100 rays
1000 rays
|